Implementing Motion Detection Inside Defined Boundaries with OpenCV
Written on
Chapter 1: Introduction to Motion Detection
In this guide, we will explore how to identify the movement of objects within a predefined boundary using OpenCV with Python. This can be applied to video footage or frames captured via a camera.
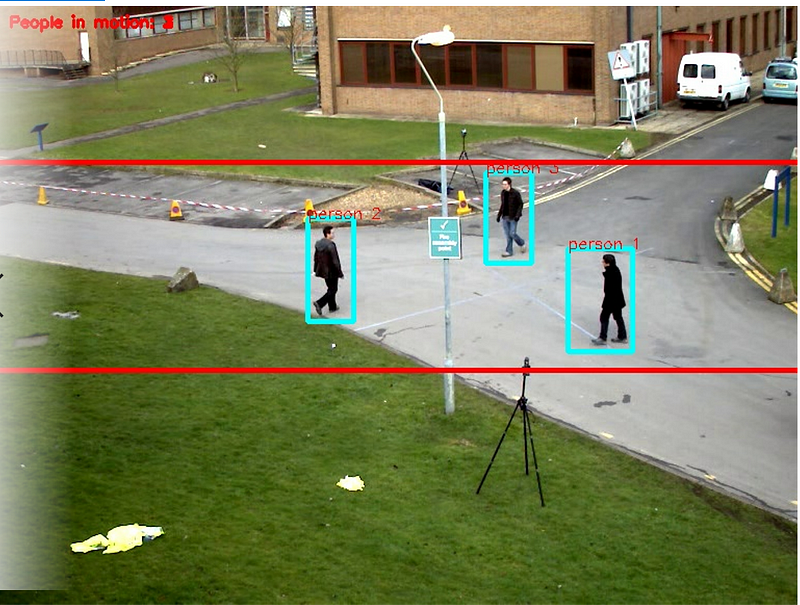
To initiate, you can acquire video input from a camera as demonstrated below:
import cv2
cap = cv2.VideoCapture(0)
if not cap.isOpened():
print("Unable to access the camera")
exit()
while True:
ret, frame = cap.read()
cv2.imshow('frame', frame)
if cv2.waitKey(1) == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
For Mac OS users, it’s essential to invoke cv2.startWindowThread() after opening a window to ensure that OpenCV can properly close the GUI displaying the frames.
Alternatively, you can work with an existing video file, reading it frame by frame as shown below:
import numpy as np
import cv2
cv2.startWindowThread()
video = cv2.VideoCapture('vtest.avi')
while video.isOpened():
ret, frame = video.read()
if not ret:
breakcv2.imshow("Video", frame)
if cv2.waitKey(1) == ord('q'):
break
video.release()
cv2.destroyAllWindows()
The VideoCapture() function allows you to capture video from either a camera or a pre-recorded file, and the video can be processed one frame at a time using read(). The read() method returns a boolean value indicating if the frame was successfully retrieved.
The waitKey() function pauses for a specified key event indefinitely. Always ensure to release the capture and destroy all windows with destroyAllWindows().
Chapter 2: Steps for Motion Detection
To detect motion in a video, follow this high-level logic:
- Read the first two consecutive frames and calculate the absolute difference between them.
- Convert the resulting image to grayscale to minimize noise.
- Smooth the grayscale image using Gaussian blur to reduce sharpness while avoiding excessive blurring.
- Segment the smoothed image by applying a threshold, turning pixels above a certain intensity white and others black.
- Use dilation to enhance the boundaries of foreground regions and remove imperfections.
- Identify contours of the moving objects within the Region of Interest (ROI).
Step 1: Capture the Initial Frames
Start by capturing the first two frames from the video and computing their absolute difference:
import numpy as np
import cv2
frame_count = 0
video = cv2.VideoCapture('vtest.avi')
ret, prev_frame = video.read()
while video.isOpened():
frame_count += 1
ret, current_frame = video.read()
if not ret:
break
frame_diff = cv2.absdiff(current_frame, prev_frame)
cv2.imshow("Absolute Diff", frame_diff)
cv2.imwrite(f"frame{frame_count}.jpg", frame_diff)
if cv2.waitKey(1) == ord('q'):
breakprev_frame = current_frame
video.release()
cv2.destroyAllWindows()
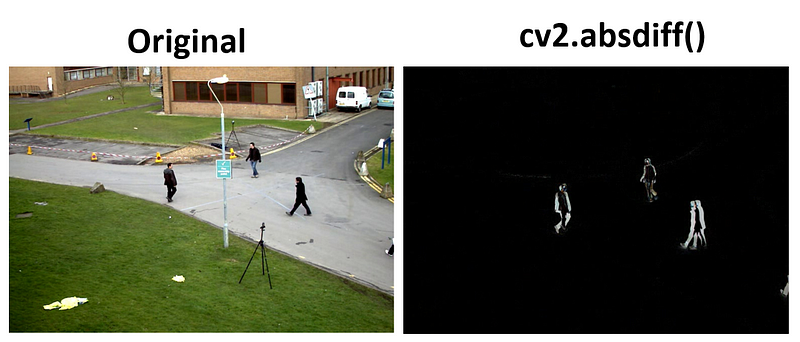
Step 2: Grayscale Conversion
Next, convert the absolute difference image to grayscale to assist in noise reduction:
gray = cv2.cvtColor(frame_diff, cv2.COLOR_BGR2GRAY)
Step 3: Image Smoothing
Smooth the grayscale image using Gaussian blur to minimize sharp edges while controlling the extent of blurring:
blur = cv2.GaussianBlur(gray, (5, 5), 0)
Step 4: Thresholding
Segment the smoothed image using OpenCV’s thresholding method:
thresh = cv2.threshold(blur, 20, 255, cv2.THRESH_BINARY)[1]
Step 5: Dilation
Apply dilation to the thresholded image to further refine the foreground regions:
dilate = cv2.dilate(thresh, None, iterations=4)

Step 6: Contour Detection
Identify contours of moving objects in the video frames.
Step 7: Filter Relevant Contours
Iterate through all contours to retain only those that are significant, ensuring they lie within the predefined Region of Interest:
# Example code for contour filtering (not provided in original content)
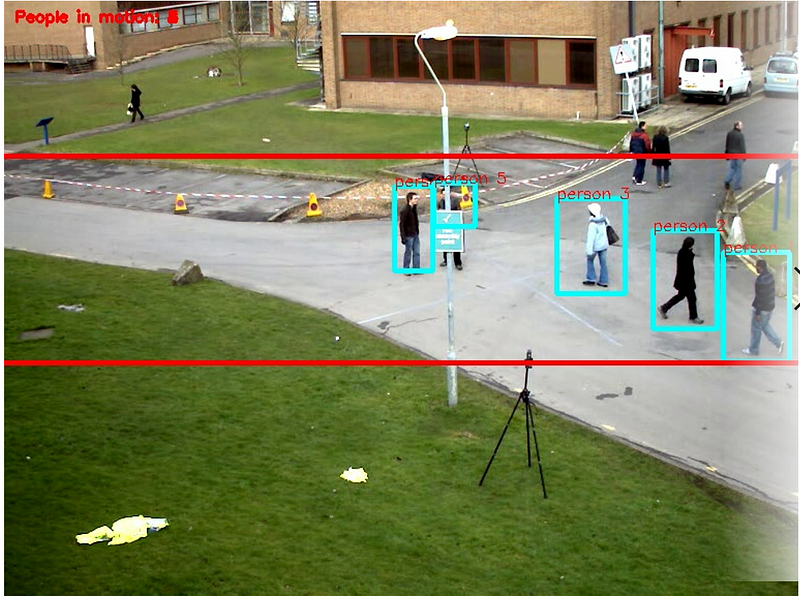
Results:
The motion detection process successfully identifies a person's movement within designated boundaries using OpenCV techniques. If the individual crosses the specified limit, the bounding box will not be drawn around them.
References:
- OpenCV: OpenCV-Python Tutorials
- Official OpenCV Documentation: docs.opencv.org
The first video demonstrates how to implement motion detection using Python and OpenCV with contours.
The second video provides a comprehensive tutorial on motion detection using OpenCV with code samples.